IAPHUB SDK
This guide will walk you through the different steps to set up the IAPHUB SDK on your app.
If you're using Adalo, follow this guide instead.
If you're using React Native, we've released a React Native UI component.
It's built on top of react-native-iaphub and aims to simplify your life by offering React components to initialize IAPHUB and display a customizable paywall translated into 6 languages.
If this solution fits your needs, please refer to this guide for setup instructions.
Install SDK
You should install the IAPHUB SDK in your app. All of our SDKs are open source and available on Github:
- iOS SDK (Swift/Objective-C) (https://github.com/iaphub/iaphub-ios-sdk)
- Android (Kotlin/Java) (https://github.com/iaphub/iaphub-android-sdk)
- React Native SDK (https://github.com/iaphub/react-native-iaphub)
- Flutter SDK (https://github.com/iaphub/flutter-iaphub)
// Install react-native-iaphub
npm install react-native-iaphub --save
// Update dependency on xcode (in the ios folder)
pod install
Our code snippets below are using the latest SDK version available. If you installed the SDK a while ago, make sure you're up to date.
Start SDK
The start method initializes IAPHUB and is a prerequisite for using other SDK methods.
It's important to note that if the start method is called again, all previous data will be reset. For instance, you'll need to authenticate the user again. Make sure to implement security measures to prevent unnecessary calls to the method.
await Iaphub.start({
appId: "5e4890f6c61fc971cf46db4d",
apiKey: "SDp7aY220RtzZrsvRpp4BGFm6qZqNkrt",
enableStorekitV2: true
});
Get the products for sale
Now, proceed to retrieve your products for sale using the getProductsForSale method.
This function will provide you with the products configured in your listing, allowing you to set up and display your paywall.
It is important to note that configuring the sandbox environment on iOS and Android is necessary to be able to test products that aren't approved yet and make test purchases for free. Otherwise the getProductsForSale method will return an empty array.
var productsForSale = await Iaphub.getProductsForSale();
Detect when the billing system is unavailable
When the billing system is unavailable, the products for sale will be filtered, and an empty array will be returned.
You can use the getBillingStatus method (after fetching the products) to detect when this is the case in order to display the appropriate error message.
On Android, we also recommend displaying an error message when the billing system is unavailable due to an outdated Google Play Store app (which can occur on older devices with outdated software). Instruct the user to open the Play Store app, navigate to "Settings," select "About," and click on "Update Play Store."
If the getBillingStatus method doesn't return any errors but your product is missing, it is most likely a misconfiguration issue.
You should check the filteredProductIds
property. If your product ID is in the array, it indicates an issue with Google Play/Apple (as we rely on them to fetch the product details).
Otherwise, it's likely an issue with IAPHUB. Please follow this guide to walk through the different possibilities that could cause this issue.
var status = await Iaphub.getBillingStatus();
if (status.error && status.error.code == "billing_unavailable") {
if (status.error.subcode == "play_store_outdated") {
// Display a message on your paywall saying that the Play Store app on the user's device is out of date, it must be updated
}
else {
// Display a message on your paywall saying that the in-app billing isn't available on the device
}
}
if (status.filteredProductIds.length) {
// Some products have been filtered because they were not returned by GooglePlay/iTunes
}
Detect when the products for sale are updated
Additionally, you can listen for the onUserUpdate event to stay informed about any updates to your products for sale.
Upon detection of an update, trigger a refresh of your data and user interface by invoking the getProductsForSale method once again.
If the cache is outdated, the SDK automatically checks if the products for sale have been updated every time the app is brought to the foreground. Therefore, when updating your products for sale, keep in mind that the changes won't take effect instantly; the cache can last for up to 24 hours.
var listener = Iaphub.addEventListener('onUserUpdate', () => {
// Refresh your data and UI here
});
Make a sandbox purchase
After successfully retrieving your products for sale, it's time to dive into your first sandbox purchase!
When a user clicks on a product available for sale, smoothly pass the product SKU to the buy method to initiate the purchase.
Please note that if you buy a product from the same subscription group as your current active subscription, it will replace the one you currently have.
try {
var transaction = await Iaphub.buy(sku);
console.log("Transaction successful: ", transaction);
}
catch (err) {
console.log(`Error: Transaction failed, message: ${err.message}, code: ${err.code}, subcode: ${err.subcode}`);
}
Webhooks (optional)
If you want to get alerts on your server when something happens, you can use webhooks.
When you do, you can check if the purchase notification has reached your server by looking at the webhookStatus property of the transaction returned by the method.
If your server responds with a '200' code, the webhookStatus property should say 'success'.
Errors
Ensure to handle any potential errors that may occur during the execution of the buy method.
Please refer to our comprehensive list of errors for guidance on how to address and manage these situations effectively.
Smart assistant
If our smart assistant detects an error stemming from a configuration issue, it will promptly notify you via email, furnishing all the essential details to rectify the problem. Additionally, a smart assistant widget will be available on the IAPHUB dashboard. Simply click on the provided link (as illustrated in the screenshot below) for further guidance and assistance.
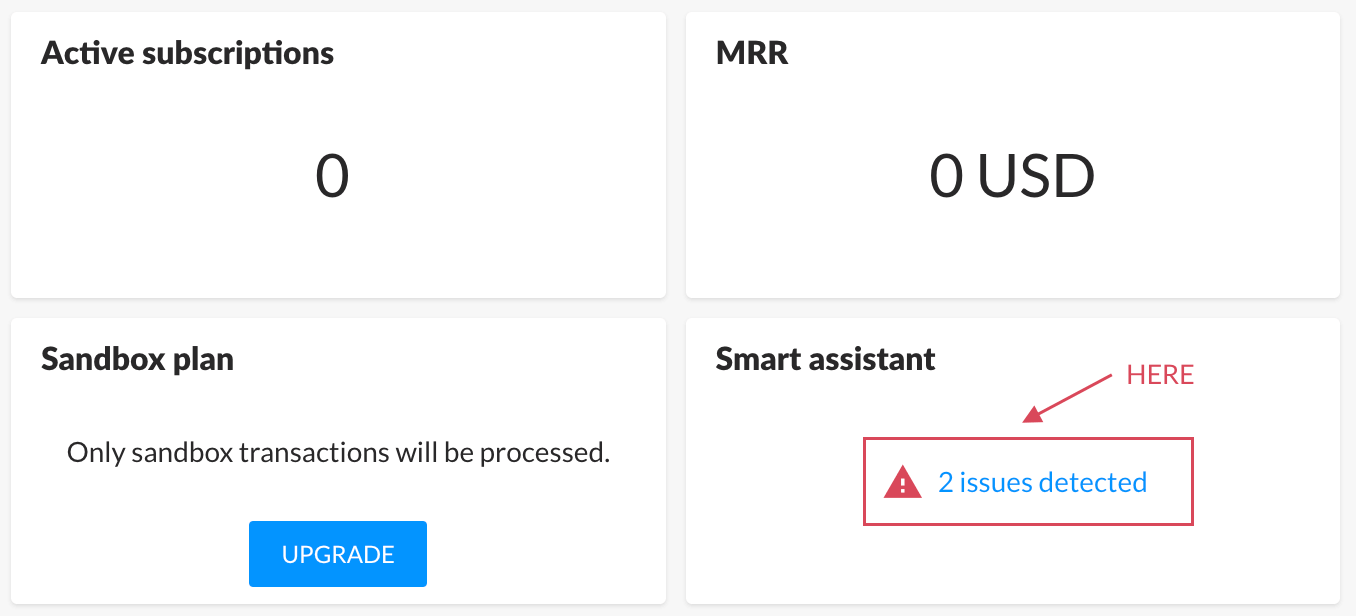
If you cannot find the cause of an error, please send us an email at [email protected]
, and we'll be happy to help.
Detect deferred purchases
It is possible for a purchase to be processed outside of the buy method. This may occur in scenarios such as:
- Making a purchase outside the app (e.g., by redeeming a promo code on the store)
- Experiencing a deferred payment (when the error code
deferred_payment
is returned by the buy method) - Encountering a payment failure due to validation issues by IAPHUB, which may succeed at a later time.
You can set up a listener to receive notifications for deferred purchases using the onDeferredPurchase event:
var listener = Iaphub.addEventListener('onDeferredPurchase', (transaction) => {
// Process deferred transaction
});
Get the active products
The getActiveProducts method retrieves all subscriptions or non-consumables owned by the user. If your app includes subscription sales, this method is essential for checking whether the user has an active subscription.
However, if you synchronize your server with IAPHUB using webhooks, you can directly query your server instead, eliminating the need for this method.
By default, the getActiveProducts method only returns subscriptions with an 'active' or 'grace_period' state.
If the includeSubscriptionStates option is specified, you can also retrieve subscriptions in a 'paused' or 'retry_period' state.
This option can be useful if you need to display a message regarding these states.
var activeProducts = await Iaphub.getActiveProducts();
Detect when the active products are updated
To stay informed about updates to your active products, use our onUserUpdate event.
This event triggers whenever a change occurs, such as a subscription being cancelled, expired, or renewed.
Upon triggering, you can refresh your data and UI by calling the getActiveProducts method again.
var listener = Iaphub.addEventListener('onUserUpdate', () => {
// Refresh your data and UI here
});
Authenticate the user (optional)
If your app has an authentication system, you can authenticate users with IAPHUB using the login method.
You should provide an id that is non-guessable and isn't public. (Email not allowed)
By authenticating your users with IAPHUB, you gain the ability to search for users on the IAPHUB dashboard using your own user ID, and through the IAPHUB API. Authenticated users can access their subscriptions from any device when logged into the same account.
If authentication with IAPHUB is not implemented, an anonymous user ID is generated on the device to identify the user. While users can still access their subscriptions on different devices, they will need to use the restore method. A subscription can be shared on up to five devices maximum after a successful restore using the same Apple/Google account.
When an anonymous user ID owns an active subscription or non-consumable product and the user is authenticated, the user accounts are merged.
This means that the active products purchased by the anonymous user ID will also become available for the authenticated user.
It's important to note that calling the login or logout method will reset the current user. Subsequently, you won't receive any onUserUpdate events until after calling getActiveProducts, getProductsForSale or getProducts.
await Iaphub.login("1e5494930c48ed07aa275fd2");
A logout method is also available to log the user out.
await Iaphub.logout();
Add a button to restore purchases
The restore method resynchronizes the purchased transactions from the user's store account (Apple/Google Play).
If a transaction has never been processed by IAPHUB, it will be returned in the newPurchases array of the response.
Additionally, if an active product (subscription/non-consumable) is already associated with another user ID, the product will seamlessly transfer to the new user and will be returned in the transferredActiveProducts array.
If you wish to disable this feature, you can conveniently manage it from the settings within the IAPHUB dashboard.
For apps lacking an authentication system, users can restore an active product (subscription/non-consumable), on up to 5 devices using the same Apple or Google account.
It's a good idea to let users know they can restore their purchases if they have any issue. You should add a visible button in your app that triggers the method. This is helpful if they ever have trouble accessing their subscriptions. By suggesting a restore, you can help them fix any issues and make sure they can use your app smoothly.
var response = await Iaphub.restore();
Test your implementation
You should, of course, always test your implementation properly to ensure everything is working correctly.
Sandbox testing is critical for testing your implementation and will allow you to purchase products without being charged. Additionally, sandbox subscriptions renew more frequently than normal subscriptions, which is beneficial for testing purposes.
Release app
With all the information we've discussed, you should find it relatively straightforward to release your app with in-app purchases using IAPHUB! Compared to developing a full custom system to monetize your app, I believe you'll appreciate the efficiency and simplicity of our solution.
If you're out of luck and your iOS app is rejected by the Apple Review Team, you should read this.
Upgrade your plan to production
Before submitting your app, remember to upgrade your IAPHUB plan to production (as shown in the screenshot below) to enable processing of real transactions.
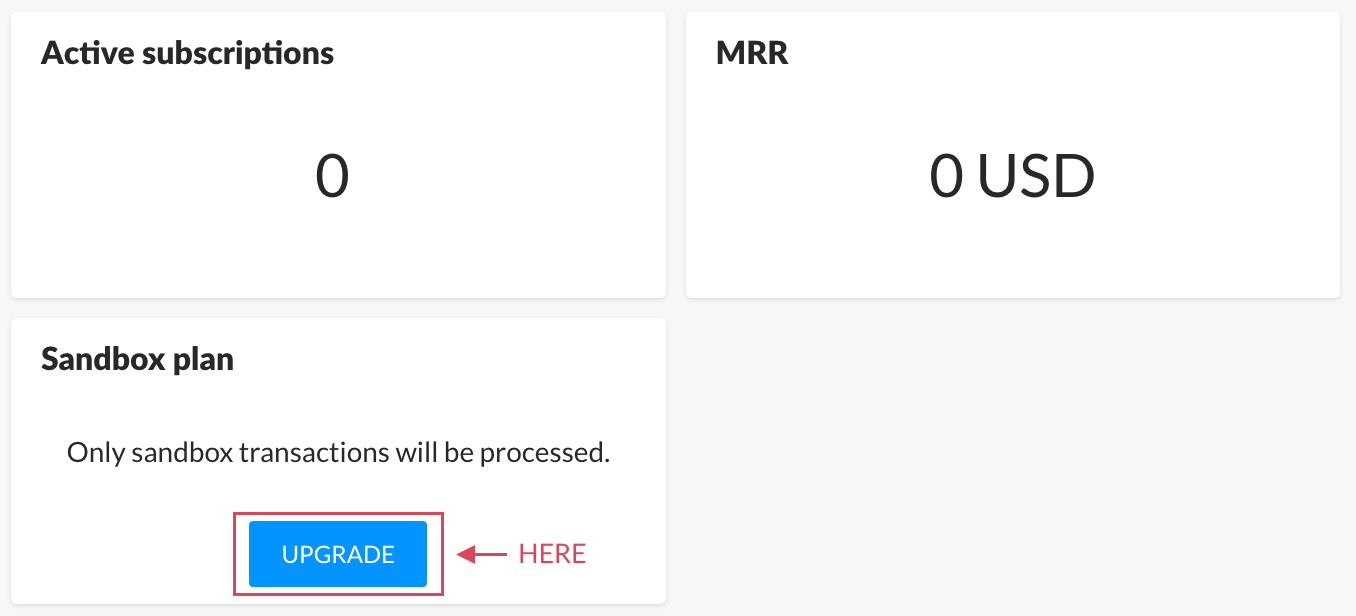
Display production data
If you want to display real transactions instead of sandbox transactions, remember to switch the selection to production data. You can find it in the top bar of the IAPHUB dashboard.
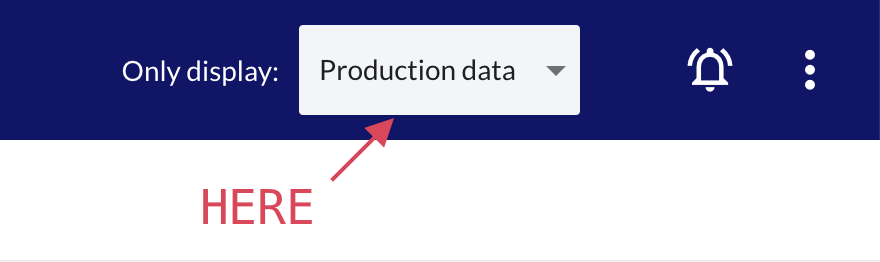
Need help?
If you encounter any issues preventing you from using the IAPHUB SDK or if you have any questions, feel free to contact us at [email protected]
.